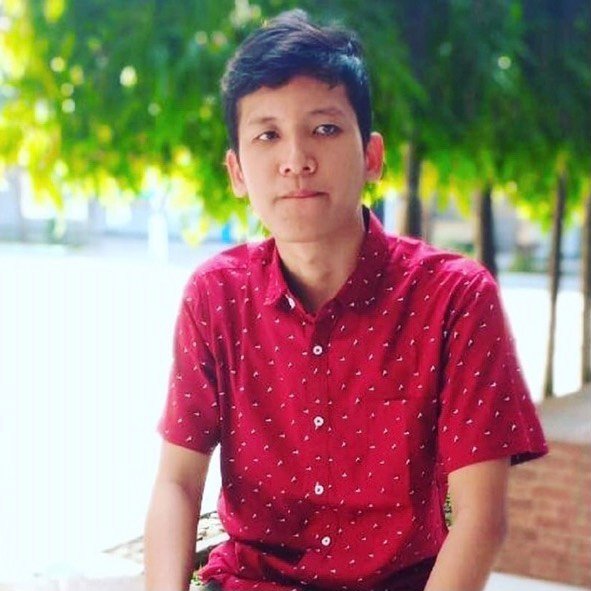
Software Engineer at Stockbit & Bibit.id
Share content with Browser Web Sharing API
The Web Sharing API has seemingly gone under the radar, It provides a way to trigger native sharing dialog of a device. It is a simple API that allows you to share text, links, files, and data to other applications installed on the device directly from a website or application.
The Web Sharing API is available in Chrome 91 and above. It is currently available on Android and Chrome OS. It is not available on iOS or desktop. You can check the browser compability here source. or meanwhile you can provide a Fallback if the API is not available. take a look at this code.
if (navigator.share) {
// Web Share API is supported
} else {
// Fallback
}
Using the Web Share API is as simple as calling the navigator.share() method and passing an object that contains at least one of the following fields:
Using the Web Share API is as simple as calling the navigator.share() method and passing an object that contains at least one of the following fields:
• url: representing the URL that want to be shared. usually this is become the document URL but you can pass any URL via Web Share API.
• title: representing the title to be shared, usually document.title
• text: Any string you want to include in the share.
if (navigator.share) {
navigator.share({
title: 'Introducing Web Share API',
url: 'https://leonardmns.dev/blog/web-share-api'
}).then(() => {
console.log('Share success!');
}).catch(console.error);
} else {
// fallback
}
once this code is being called with the supported browser it will trigger the native sharing dialog of the device. Target share can be social media apps, email, SMS, Instant Messaging, and more.
Web Share API is promised based function so you can attach .then() method perhaps you want to do something after the share is successful. You can also attach .catch() method to handle the error.
Provide a Fallback!
If the Web Share API is not available you can provide a fallback. You can use navigator.clipboard to copy the URL to the clipboard. You can also do anything inside the else scope. this is to make sure that the user can still share the content even if the Web Share API is not available.
if (navigator.share) {
...
} else {
// fallback
navigator.clipboard.writeText('https://leonardmns.dev/blog/web-share-api');
}
This covers pretty much the baseline for what you need to know about the Web Share API. I hope this article helps you to understand the Web Share API. If you have any questions or suggestions please feel free to ask me, happy coding 💖