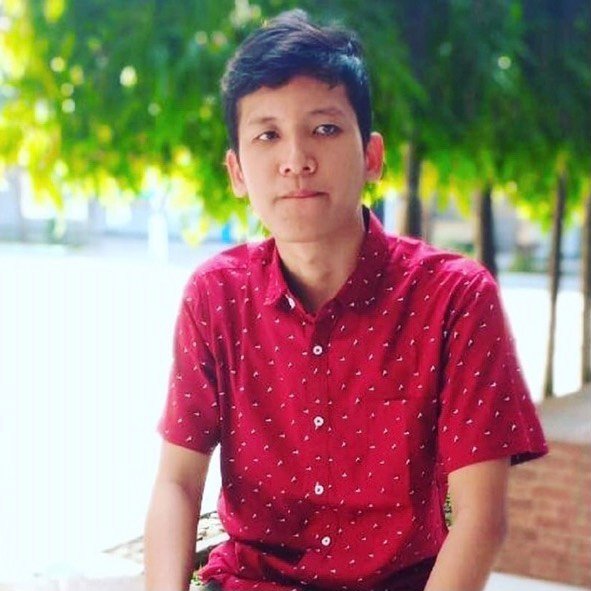
Software Engineer at Stockbit & Bibit.id
Cut expensive computation call with redis! 🚀
Did you ever thinking about how you can cache your response or you don’t want to do some expensive computation everytime the request got called 🤔 then redis might come handy to help you solving the problem.
First let install redis and then run it in the same address within your network otherwise it won’t work as expected. in this example i will use homebrew to install redis. From the terminal run this command:
brew install redis
To test your Redis installation, you can run the redis-server executable from the command line:
redis-server
If successful, you’ll see the startup logs for Redis, and Redis will be running in the foreground. And then let’s head back to our source code and start with importing the redis client, you can get the redis client from npmjs.com. or use your own package manager like npm, yarn, pnpm, etc.
import * as redis from "redis";
/**
* @this connect_redis_client
*/
const redisClient: redis.RedisClientType<any, any> = redis.createClient({});
redisClient.connect();
After that let’s store our first data to redis, in this example i’m using weapon/skinlevels as the key of the value, you can use any key you want. and then we set the TTL time to live in this example i set the TTL to 1 day. so the data will be removed from redis after 1 day passed.
One thing you need to remember that redis can only store a string, so if you want to store a complex data structure like an array or object you need to convert it to a string first before storing into redis memory.
public static getWeaponSkinLevels = async (): Promise<any> => {
// let say this is an expensive calls
const response = await ApiRequest({
baseurl: "https://valorant-api.com/v1/",
path: "weapons/skinlevels",
method: "GET",
});
if (response) {
redisClient.setEx("weapon/skinlevels", 60 * 60 * 24, JSON.stringify(response.data));
return response.data;
}
throw new Error("No data found");
};
After we have our first data stored into redis, let’s take a look of example how to get the data from redis or how to use it as a cookie in your request so you can get the data from redis without doing any expensive computation on every request.
public static getWeaponSkinLevels = async (): Promise<any> => {
const data: string | null = await redisClient.get("weapons/skinlevels");
if (data) {
return JSON.parse(data);
}
// let say this is an expensive calls
const response = await ApiRequest({
baseurl: "https://valorant-api.com/v1/",
path: "weapons/skinlevels",
method: "GET",
});
if (response) {
redisClient.setEx("weapon/skinlevels", 60 * 60 * 24, JSON.stringify(response.data));
return response.data;
}
throw new Error("No data found");
};
That’s it!, and then your expensive calls only happen once in a day, but make sure you do this only to the data that is not being update frequently otherwise your user will get older data.
Also you can use redis for many usage other than this this, ex doing email verification, rate limitting your API, etc. if you have any question or suggestion please feel free to leave me a message and i’ll see you guys in the next tutorial. happy coding 💖